Table of Contents In Laravel, many-to-many relationships are established using an intermediate table that holds foreign keys from the related
Unlocking the Power of One-to-Many Relationships in Laravel Expert Tips and Real-Life Examples for Improved Performance
- Muhammad Waqas
- May 15, 2023
- 5:04 am
- No Comments
Table of Contents
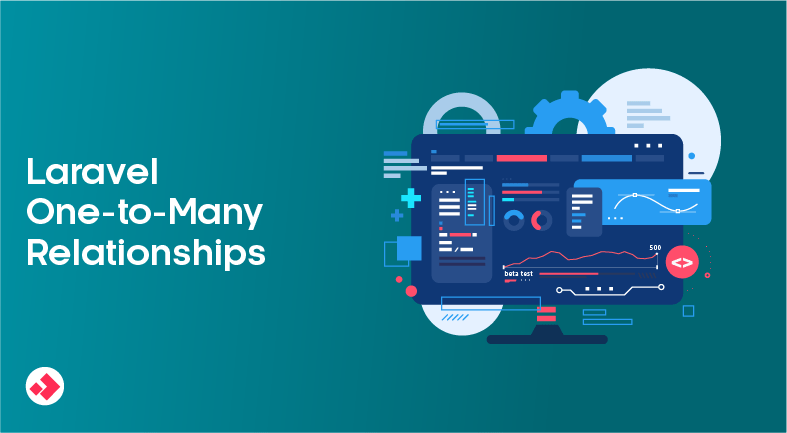
In Laravel, relationships are one of the core concepts that make working with databases and models much more straightforward. One of the most common types of relationships is the one-to-many relationship, also known as the inverse or belongs-to relationship. In this blog post, we’ll explore what this type of relationship is, how it works in Laravel, and provide three real-life examples of how you can use it.
What is a One-to-Many (Inverse) / Belongs-To Relationship?
A one-to-many relationship is a type of database relationship where one record in a table has many related records in another table. In Laravel, this is typically referred to as the inverse or belongs-to relationship because the related records belong to the record in the other table.
For example, let’s say we have two tables: users
and posts
. Each user can have multiple posts, but each post belongs to only one user. In this case, we would define a one-to-many relationship between the users
and posts
tables, where the posts
table “belongs to” the users
table.
How does the One-to-Many (Inverse) / Belongs-To Relationship work in Laravel?
In Laravel, we can define a one-to-many relationship using the belongsTo
and hasMany
methods. The belongsTo
method defines the relationship on the “belongs to” side of the relationship, while the hasMany
method defines the relationship on the “has many” side of the relationship.
Let’s use the example of the users
and posts
tables from earlier. We would define the relationship like this:
// In the User model
public function posts()
{
return $this->hasMany(Post::class);
}
// In the Post model
public function user()
{
return $this->belongsTo(User::class);
}
In this example, the posts
method on the User
model defines that a user can have many posts, while the user
method on the Post
model defines that a post belongs to a user.
To use this relationship in our application, we can use eager loading to load all related records at once, rather than querying the database for each related record individually. We’ll cover this in more detail in the examples below.
Example 1: Blog Posts and Comments
Let’s say we have a blog with posts and comments. Each post can have many comments, but each comment belongs to only one post. We can define the relationship like this:
// In the Post model
public function comments()
{
return $this->hasMany(Comment::class);
}
// In the Comment model
public function post()
{
return $this->belongsTo(Post::class);
}
Now, to load all comments for a post, we can use eager loading like this:
$post = Post::with('comments')->find(1);
This will load the post with ID 1 and all related comments in a single query, rather than querying the database for each comment individually.
Example 2: Customers and Orders
Let’s say we have an e-commerce site with customers and orders. Each customer can have many orders, but each order belongs to only one customer. We can define the relationship like this:
// In the Customer model
public function orders()
{
return $this->hasMany(Order::class);
}
// In the Order model
public function customer()
{
return $this->belongsTo(Customer::class);
}
Now, to load all orders for a customer, we can use eager loading like this:
$customer = Customer::with('orders')->find(1);
This will load the customer with ID 1 and all related orders in a single query, rather than querying the database for each order individually.
Example 3: Teachers and Classes
Let’s say we have a school with teachers and classes. Each teacher can teach many classes, but each class has only one teacher. We can define the relationship like this:
// In the Teacher model
public function classes()
{
return $this->hasMany(Class::class);
}
// In the Class model
public function teacher()
{
return $this->belongsTo(Teacher::class);
}
Now, to load all classes for a teacher, we can use eager loading like this:
$teacher = Teacher::with('classes')->find(1);
This will load the teacher with ID 1 and all related classes in a single query, rather than querying the database for each class individually.
Conclusion
The one-to-many (inverse) / belongs-to relationship is a powerful tool in Laravel that allows us to easily define and work with related records in our database. By using eager loading, we can efficiently load all related records in a single query, which can greatly improve the performance of our application. In this blog post, we’ve covered what this relationship is, how it works in Laravel, and provided three real-life examples of how you can use it in your own applications.
Popular Post
Table of Contents Introduction: Laravel is a PHP framework that has become quite popular in recent years. One of the
Table of Contents In Laravel, models are the backbone of the application. They act as a bridge between the database
Table of Contents Laravel is a popular PHP framework that is widely used for web application development. One of the
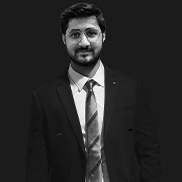
An experienced Code Debugger with a strong track record of identifying and resolving complex software issues. I aim to contribute my analytical skills and expertise in debugging tools to ensure efficient and reliable software operations and deliver high-quality software products that meet customer requirements.
If you found this content valuable, please show your support by following me on Medium, LinkedIn, Twitter and GitHub. Your support will motivate me to create more informative content in the future. Don’t forget to give a clap or share this blog with others if you found it helpful!