Table of Contents In Laravel, many-to-many relationships are established using an intermediate table that holds foreign keys from the related
Unleashing the Power of Intermediate Tables in Laravel: Tips and Tricks for Easy Data Retrieval
- Muhammad Waqas
- May 15, 2023
- 4:55 am
- No Comments
Table of Contents
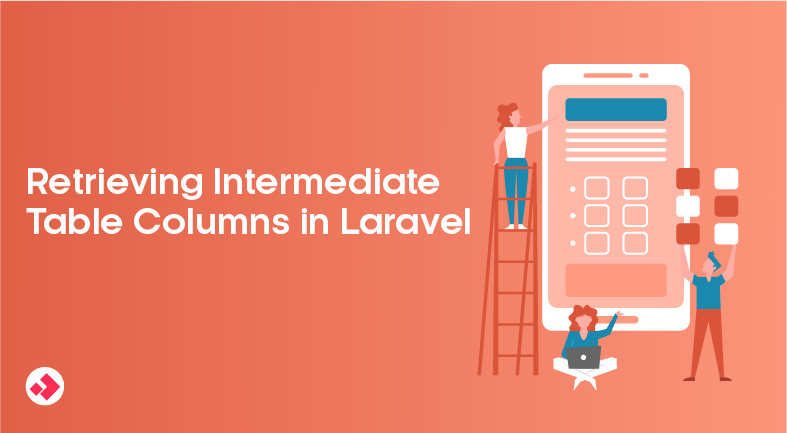
Creating the Database Table and Models:
In Laravel, intermediate tables are used to represent many-to-many relationships between database tables. For example, if you have a “users” table and a “roles” table, and each user can have multiple roles and each role can be assigned to multiple users, you would use an intermediate table called “role_user” to represent this relationship.
Defining the Relationships in the Models
Sometimes, you may need to retrieve data from the intermediate table, such as when you want to display a list of users and their roles. In this blog post, we will explore how to retrieve intermediate table columns in Laravel.
Ifyou don’t know How to Define Custom Intermediate Table Models, please read our previous blog post on the topic: 🔗📚🌐 Many-to-Many Relationships in Laravel: How to Define Custom Intermediate Table Models 🤝💻
First, let’s create the necessary database tables and models. We’ll use the example of a “users” table and a “roles” table with a many-to-many relationship:
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->timestamps();
});
Schema::create('roles', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->timestamps();
});
Schema::create('role_user', function (Blueprint $table) {
$table->unsignedBigInteger('role_id');
$table->unsignedBigInteger('user_id');
$table->timestamps();
$table->foreign('role_id')->references('id')->on('roles');
$table->foreign('user_id')->references('id')->on('users');
});
Next, let’s define the relationships in our models. In the User model:
public function roles()
{
return $this->belongsToMany(Role::class);
}
And in the Role model:
public function users()
{
return $this->belongsToMany(User::class);
}
Retrieving Multiple Columns from the Pivot Table
Now, let’s say we want to retrieve the “created_at” column from the intermediate “role_user” table for a specific user. We can do this by chaining the “withPivot” method onto our “belongsToMany” relationship in the User model:
$user = User::find(1);
$roles = $user->roles()->withPivot('created_at')->get();
foreach ($roles as $role) {
echo $role->pivot->created_at;
}
In this example, we’re retrieving the roles for the user with an ID of 1, and using the “withPivot” method to include the “created_at” column from the “role_user” table. We then loop through the roles and output the “created_at” value from the pivot table for each role.
You can also retrieve multiple columns from the pivot table by passing an array of column names to the “withPivot” method:
$roles = $user->roles()->withPivot(['created_at', 'updated_at'])->get();
Conclusion
In conclusion, retrieving intermediate table columns in Laravel is easy using the “withPivot” method on your “belongsToMany” relationship. By including the desired columns from your pivot table, you can access and use this data in your application as needed.
Popular Post
Table of Contents Introduction: Laravel is a PHP framework that has become quite popular in recent years. One of the
Table of Contents In Laravel, models are the backbone of the application. They act as a bridge between the database
Table of Contents Laravel is a popular PHP framework that is widely used for web application development. One of the
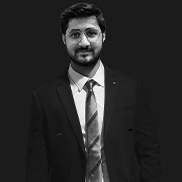
An experienced Code Debugger with a strong track record of identifying and resolving complex software issues. I aim to contribute my analytical skills and expertise in debugging tools to ensure efficient and reliable software operations and deliver high-quality software products that meet customer requirements.
If you found this content valuable, please show your support by following me on Medium, LinkedIn, Twitter and GitHub. Your support will motivate me to create more informative content in the future. Don’t forget to give a clap or share this blog with others if you found it helpful!