Table of Contents In Laravel, many-to-many relationships are established using an intermediate table that holds foreign keys from the related
Streamline Your Laravel Development with Route Model Binding A Real-Life Example
- Muhammad Waqas
- May 15, 2023
- 5:08 am
- No Comments
Table of Contents
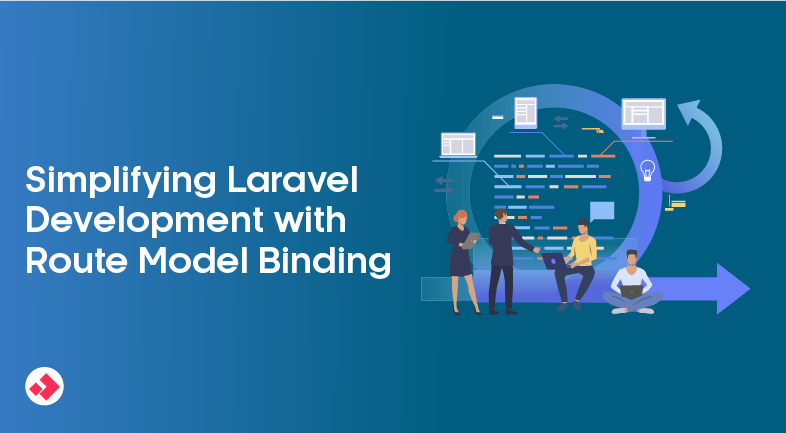
Laravel is a popular PHP framework that makes it easy to build web applications quickly and efficiently. One of the key features of Laravel is its routing system, which allows developers to define the URLs that their application responds to and the actions that should be taken when those URLs are requested.
Route model binding is a feature of Laravel’s routing system that allows developers to automatically inject model instances into their controllers based on the value of a route parameter. In this blog post, we’ll explore how route model binding works and provide a real-life example of how it can be used to simplify the development of a web application.
Understanding Route Model Binding:
Route model binding is a technique for automatically injecting instances of a model into a controller method. This is done by matching a route parameter with a column in the database table associated with the model. When a request is made to a URL that includes a parameter that matches the value of the column in the database, Laravel will automatically retrieve the corresponding model instance and pass it to the controller method.
To use route model binding in Laravel, you need to define a route parameter and specify the name of the associated model class in the route definition. For example, if you have a model named “Product” and you want to retrieve an instance of that model based on a URL parameter named “id”, you would define your route like this:
Route::get('/products/{product}', 'ProductController@show');
In this example, the “product” parameter in the URL will be matched with the “id” column in the “products” database table. When a request is made to this URL, Laravel will automatically retrieve the corresponding product instance and pass it to the “show” method of the “ProductController” class.
Using Route Model Binding in a Real-Life Example:
To see how route model binding can simplify the development of a web application, let’s consider an example of an e-commerce site that sells products. We want to display a list of products on the homepage and allow users to click on a product to see more details.
Traditionally, we would have to manually retrieve the product instance from the database in the controller method for the product details page. We would need to write code like this:
public function show($id)
{
$product = Product::find($id);
return view('products.show', ['product' => $product]);
}
With route model binding, however, we can simplify this code significantly. We can define our route like this:
And our controller method can look like this:
Route::get('/products/{product}', 'ProductController@show');
public function show(Product $product)
{
return view('products.show', ['product' => $product]);
}
In this example, Laravel will automatically retrieve the product instance from the database based on the “id” column and pass it to the controller method. We can then use the $product variable in our view to display the product details.
By using route model binding, we’ve eliminated the need to manually retrieve the product instance from the database in our controller method. This saves us time and reduces the amount of code we need to write, making our application easier to maintain and less error-prone.
Conclusion:
Route model binding is a powerful feature of Laravel’s routing system that can simplify the development of web applications. By automatically injecting model instances into our controller methods based on route parameters, we can reduce the amount of code we need to write and make our applications more efficient and easier to maintain. Whether you’re building a simple blog or a complex e-commerce site, route model binding is a technique that can save you time and effort in your development process.
Popular Post
Table of Contents Introduction: Laravel is a PHP framework that has become quite popular in recent years. One of the
Table of Contents In Laravel, models are the backbone of the application. They act as a bridge between the database
Table of Contents Laravel is a popular PHP framework that is widely used for web application development. One of the
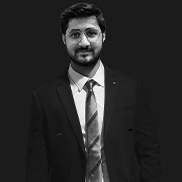
An experienced Code Debugger with a strong track record of identifying and resolving complex software issues. I aim to contribute my analytical skills and expertise in debugging tools to ensure efficient and reliable software operations and deliver high-quality software products that meet customer requirements.
If you found this content valuable, please show your support by following me on Medium, LinkedIn, Twitter and GitHub. Your support will motivate me to create more informative content in the future. Don’t forget to give a clap or share this blog with others if you found it helpful!