Table of Contents In Laravel, many-to-many relationships are established using an intermediate table that holds foreign keys from the related
Products CRUD Example In Laravel 10 Industry Best Practice
- Muhammad Waqas
- May 15, 2023
- 5:09 am
- No Comments
Table of Contents
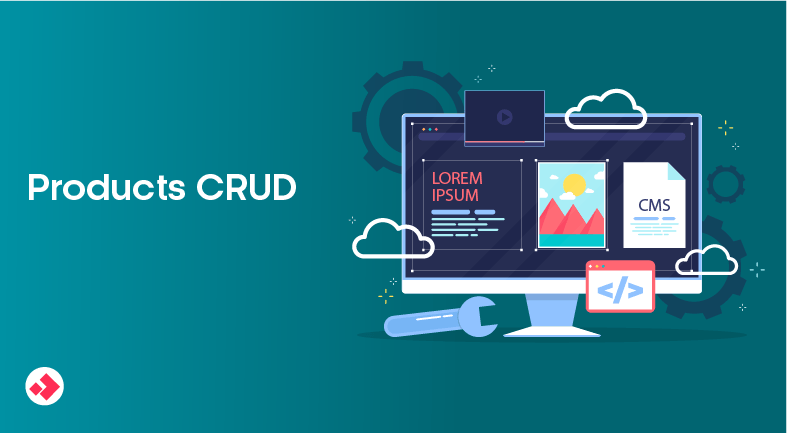
Introduction:
Laravel is a PHP framework that has become quite popular in recent years. One of the reasons for its popularity is that it follows industry best practices, including the use of Model-View-Controller (MVC) architecture, which separates business logic, presentation, and data handling. In this blog post, we will be creating a simple example of CRUD (Create, Read, Update, Delete) operations for a Product model using Laravel’s industry best practices.
We will be using Laravel 10 for this example. Let’s get started.
Step 1: Create a new Laravel project
Create a new Laravel project using the following command:
composer create-project --prefer-dist laravel/laravel product-crud-example
Step 2: Create a Product model
In Laravel, a model represents a database table. We will create a Product
model to represent our products table.
php artisan make:model Product -mcr
This command will create a Product
model with the following files:
app/Models/Product.php
database/migrations/2023_03_25_000000_create_products_table.php
app/Http/Controllers/ProductController.php
routes/web.php
The -mcr
option will also create a migration, controller, and resourceful routes for the Product
model.
Step 3: Define the products table schema
Open database/migrations/2023_03_25_000000_create_products_table.php
file and define the schema for the products table.
id();
$table->string('name');
$table->text('description');
$table->decimal('price', 8, 2);
$table->timestamps();
});
}
public function down(): void
{
Schema::dropIfExists('products');
}
}
This schema defines the id
, name
, description
, price
, and timestamps
columns for the products
table.
Step 4: Define the Product model
Open the app/Models/Product.php
file and define the Product
model.
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Product extends Model
{
use HasFactory;
protected $fillable = [
'name',
'description',
'price',
];
}
The Product
model extends the base Model
class and uses the HasFactory
trait. The $fillable
property defines which model attributes are mass assignable.
Step 5: Define Custom Request Classes
Create a new StoreProductRequest class using the following command:
php artisan make:request StoreProductRequest
Open the app/Http/Requests/StoreProductRequest.php
file and define the StoreProductRequest
.
'required|string|max:255',
'description' => 'required|string',
'price' => 'required|numeric|min:0',
];
}
}
Create a new UpdateProductRequest class using the following command:
php artisan make:request UpdateProductRequest
Open the app/Http/Requests/UpdateProductRequest.php
file and define the UpdateProductRequest
.
'required|string|max:255',
'description' => 'required|string',
'price' => 'required|numeric|min:0',
];
}
}
Step 6: Define the Product controller
Open the app/Http/Controllers/ProductController.php
file and define the ProductController
.
validated());
return redirect()->route('products.index');
}
/**
* Show the form for editing the specified resource.
*
* @param Product $product
* @return View
*/
public function edit(Product $product): View
{
return view('products.edit', compact('product'));
}
/**
* Update the specified resource in storage.
*
* @param UpdateProductRequest $request
* @param Product $product
* @return RedirectResponse
*/
public function update(UpdateProductRequest $request, Product $product): RedirectResponse
{
$product->update($request->validated());
return redirect()->route('products.index');
}
/**
* Remove the specified resource from storage.
*
* @param Product $product
* @return RedirectResponse
*/
public function destroy(Product $product): RedirectResponse
{
$product->delete();
return redirect()->route('products.index');
}
}
Step 7: Define the Product Views
Create the `products` directory inside the `resources/views` directory. Create the following views inside the `products` directory:
create files:
– index.blade.php
– create.blade.php
– edit.blade.php
index.blade.php
@extends('layouts.app')
@section('content')
Products
Name
Description
Price
Actions
@foreach ($products as $product)
{{ $product->name }}
{{ $product->description }}
{{ $product->price }}
Edit
@csrf
@method('DELETE')
@endforeach
@endsection
The index.blade.php
view displays a table with all the products in the database. It also provides links to create a new product and edit or delete existing products.
create.blade.php
@extends('layouts.app')
@section('content')
Create Product
@csrf
@endsection
The create.blade.php
view displays a form to create a new product. It includes fields for the name, description, and price of the product.
edit.blade.php
@extends('layouts.app')
@section('content')
Edit Product
@csrf
@method('PATCH')
name) }}" required>
price) }}" step="0.01" required>
@endsection
The edit.blade.php
view displays a form to edit an existing product. It pre-populates the fields with the current values of the product and updates the product in the database when the form is submitted.
Step 8: Define the product routes
Route::get('/products', [ProductController::class, 'index'])->name('products.index');
Route::get('/products/create', [ProductController::class, 'create'])->name('products.create');
Route::post('/products', [ProductController::class, 'store'])->name('products.store');
Route::get('/products/{product}/edit', [ProductController::class, 'edit'])->name('products.edit');
Route::patch('/products/{product}', [ProductController::class, 'update'])->name('products.update');
Route::delete('/products/{product}', [ProductController::class, 'destroy'])->name('products.destroy');
These routes map the HTTP methods and URIs to the methods defined in the ProductController
class.
Step 9: Run Project
php artisan serve
you get something like this:
INFO Server running on [http://127.0.0.1:8000].
Conclusion:
In this blog post, we have learned how to implement the CRUD operations for a Product
model in Laravel. We have followed the industry best practices to create a Request class to validate the input, a Model class to represent the database table, a Controller class to handle the HTTP requests, and views to display and process the input. We have also defined the routes to map the HTTP methods and URIs to the methods in the controller. This is just a basic example, but it can be extended and customized to fit your specific needs.
Popular Post
Table of Contents Introduction: Laravel is a PHP framework that has become quite popular in recent years. One of the
Table of Contents In Laravel, models are the backbone of the application. They act as a bridge between the database
Table of Contents Laravel is a popular PHP framework that is widely used for web application development. One of the
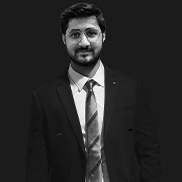
An experienced Code Debugger with a strong track record of identifying and resolving complex software issues. I aim to contribute my analytical skills and expertise in debugging tools to ensure efficient and reliable software operations and deliver high-quality software products that meet customer requirements.
If you found this content valuable, please show your support by following me on Medium, LinkedIn, Twitter and GitHub. Your support will motivate me to create more informative content in the future. Don’t forget to give a clap or share this blog with others if you found it helpful!