Table of Contents In Laravel, many-to-many relationships are established using an intermediate table that holds foreign keys from the related
Mastering the Model Lifecycle in Laravel A Comprehensive Guide
- Muhammad Waqas
- May 15, 2023
- 5:09 am
- No Comments
Table of Contents
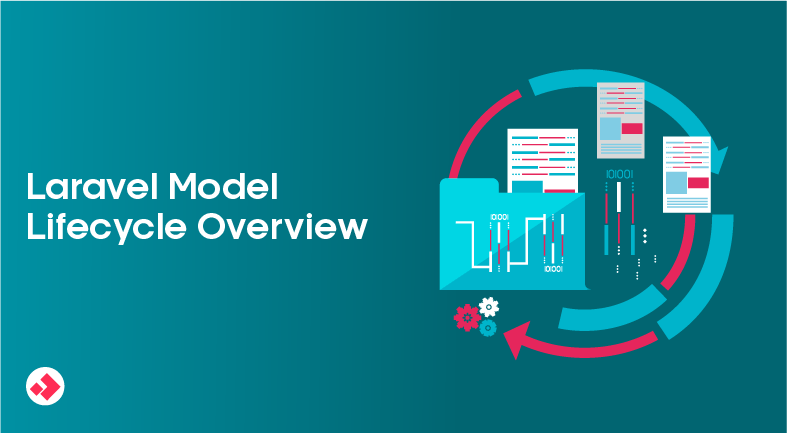
In Laravel, models are the backbone of the application. They act as a bridge between the database and the application, allowing developers to interact with the database in a more intuitive way. The model lifecycle in Laravel refers to the various stages that a model goes through during its lifetime, from creation to deletion. Understanding the model lifecycle is crucial to building robust and scalable applications in Laravel.
Here are the various stages in the model lifecycle in Laravel:
- Creating a Model
- Retrieving Models
- Updating Models
- Deleting Models
Let’s dive deeper into each stage and understand how they work in Laravel.
Creating a Model:
Creating a model in Laravel involves defining a class that extends the Illuminate\Database\Eloquent\Model
class. The class should also have a table property that specifies the database table associated with the model. Here’s an example:
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $table = 'users';
}
Once the model is defined, you can create a new instance of it and save it to the database using the create
method:
$user = User::create([
'name' => 'John Doe',
'email' => 'johndoe@example.com',
]);
This will create a new record in the users
table with the specified data.
Retrieving Models:
Retrieving models in Laravel is easy and can be done using the get
method on the model class. Here’s an example:
$users = User::get();
This will retrieve all the records from the users
table and return them as a collection of User
objects.
You can also retrieve a single record using the find
method:
$user = User::find(1);
This will retrieve the record with the primary key value of 1 from the users
table and return it as a User
object.
Updating Models:
Updating models in Laravel involves retrieving a model from the database, updating its properties, and then saving the changes back to the database. Here’s an example:
$user = User::find(1);
$user->name = 'Jane Doe';
$user->save();
This will retrieve the user with the primary key value of 1, update the name
property to ‘Jane Doe’, and save the changes back to the database.
You can also update multiple records at once using the update
method:
User::where('age', '>', 18)->update(['status' => 'active']);
This will update all the records in the users
table where the age
column is greater than 18 and set their status
column to ‘active’.
Deleting Models:
Deleting models in Laravel involves retrieving a model from the database and then calling the delete
method on it. Here’s an example:
$user = User::find(1); $user->delete();
This will retrieve the user with the primary key value of 1 and delete it from the database.
You can also delete multiple records at once using the delete
method:
User::where('age', 'delete();
This will delete all the records in the users
table where the age
column is less than 18.
Conclusion:
Understanding the model lifecycle in Laravel is crucial to building robust and scalable applications. By following the examples above, you can create, retrieve, update, and delete models in your Laravel application with ease.
Popular Post
Table of Contents Introduction: Laravel is a PHP framework that has become quite popular in recent years. One of the
Table of Contents In Laravel, models are the backbone of the application. They act as a bridge between the database
Table of Contents Laravel is a popular PHP framework that is widely used for web application development. One of the
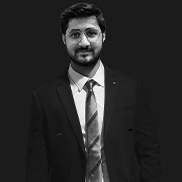
An experienced Code Debugger with a strong track record of identifying and resolving complex software issues. I aim to contribute my analytical skills and expertise in debugging tools to ensure efficient and reliable software operations and deliver high-quality software products that meet customer requirements.
If you found this content valuable, please show your support by following me on Medium, LinkedIn, Twitter and GitHub. Your support will motivate me to create more informative content in the future. Don’t forget to give a clap or share this blog with others if you found it helpful!