Table of Contents In Laravel, many-to-many relationships are established using an intermediate table that holds foreign keys from the related
Many-to-Many Relationships in Laravel: How to Define Custom Intermediate Table Models
- Muhammad Waqas
- May 15, 2023
- 5:00 am
- No Comments
Table of Contents
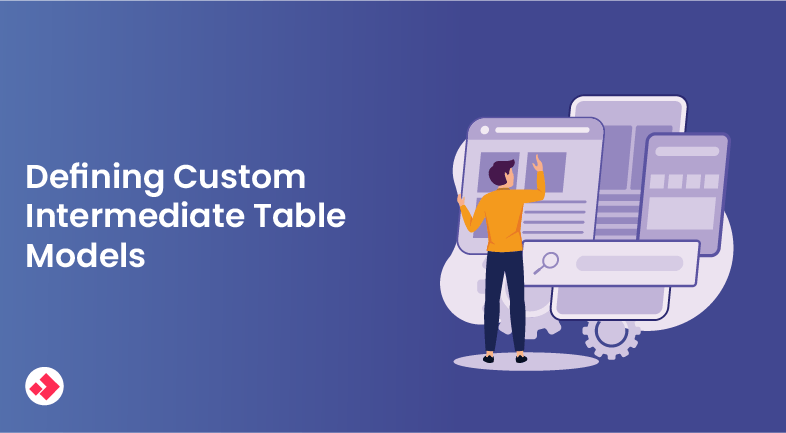
Laravel is a popular PHP framework that offers a lot of features out of the box to make it easy to build robust web applications. One such feature is the ability to define custom intermediate table models. In this blog, we will take a look at what intermediate table models are and how to define custom ones in Laravel.
Ifyou don’t know how many-to-many relationships work, please read our previous blog post on the topic: “Many-to-Many Relationships in Laravel: Connecting Your Data with Ease”
What are Intermediate Table Models?
Intermediate tables are database tables that are used to link two other tables that have a many-to-many relationship. For example, consider a blog system where each blog post can have many tags, and each tag can be applied to many blog posts. In this case, we would have three tables:
- blog_posts
- tags
- blog_post_tag (an intermediate table that links blog_posts and tags)
Laravel’s Eloquent ORM provides a way to work with many-to-many relationships by defining a “belongsToMany” relationship between two models. However, in some cases, we might want to add additional functionality to this relationship. For example, we might want to store additional data in the intermediate table or perform additional validation on the data before it is saved. This is where intermediate table models come in.
Intermediate table models are Eloquent models that represent the intermediate table in a many-to-many relationship. By defining a custom intermediate table model, we can add additional functionality to the relationship between two models.
Defining a Custom Intermediate Table Model
To define a custom intermediate table model in Laravel, we need to follow a few simple steps:
- Create a new model that extends the “Pivot” class.
- Define the table name for the intermediate table in the new model.
- Define any additional columns or relationships that we want to add to the intermediate table.
Let’s take a look at an example. Suppose we have the following many-to-many relationship between User and Role models:
class User extends Model
{
public function roles()
{
return $this->belongsToMany(Role::class);
}
}
class Role extends Model
{
public function users()
{
return $this->belongsToMany(User::class);
}
}
By default, Laravel will use the “user_role” table as the intermediate table for this relationship. Suppose we want to add a “status” column to this table to represent whether the user’s role is active or inactive. To do this, we can define a custom intermediate table model as follows:
use Illuminate\Database\Eloquent\Relations\Pivot;
class UserRole extends Pivot
{
protected $table = 'user_role';
public function user()
{
return $this->belongsTo(User::class);
}
public function role()
{
return $this->belongsTo(Role::class);
}
}
In this example, we have defined a new model called UserRole that extends the Pivot class. We have also defined the table name for the intermediate table as “user_role”. Finally, we have added two new belongsTo relationships to represent the User and Role models.
With this custom intermediate table model, we can now use it in our many-to-many relationship definition:
class User extends Model
{
public function roles()
{
return $this->belongsToMany(Role::class)
->using(UserRole::class)
->withPivot('status');
}
}
In this example, we are using the “using” method to specify the custom intermediate table model, and the “withPivot” method to include the “status” column in the pivot table.
Conclusion
In this blog, we have learned how to define custom intermediate table models in Laravel. By creating a custom intermediate table model, we can add additional functionality to many-to-many relationships, such as storing additional data in the intermediate table or performing.
Popular Post
Table of Contents Introduction: Laravel is a PHP framework that has become quite popular in recent years. One of the
Table of Contents In Laravel, models are the backbone of the application. They act as a bridge between the database
Table of Contents Laravel is a popular PHP framework that is widely used for web application development. One of the
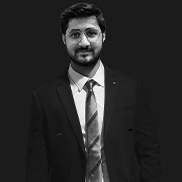
An experienced Code Debugger with a strong track record of identifying and resolving complex software issues. I aim to contribute my analytical skills and expertise in debugging tools to ensure efficient and reliable software operations and deliver high-quality software products that meet customer requirements.
If you found this content valuable, please show your support by following me on Medium, LinkedIn, Twitter and GitHub. Your support will motivate me to create more informative content in the future. Don’t forget to give a clap or share this blog with others if you found it helpful!