Table of Contents In Laravel, many-to-many relationships are established using an intermediate table that holds foreign keys from the related
Many-to-Many Relationships in Laravel: Connecting Your Data with Ease!
- Muhammad Waqas
- May 15, 2023
- 5:02 am
- No Comments
Table of Contents
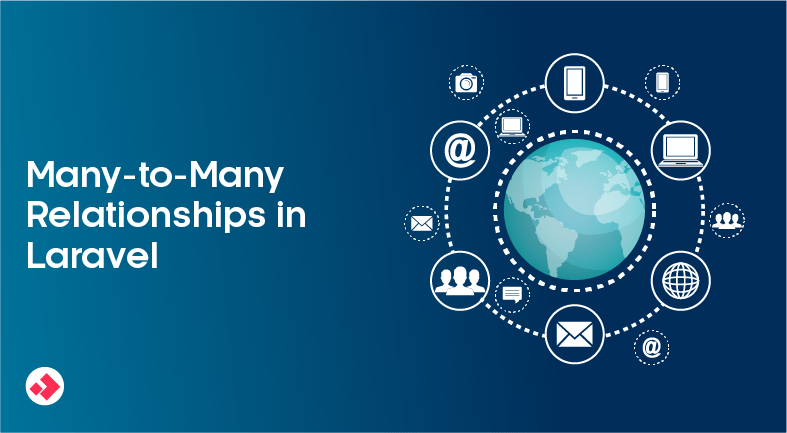
In Laravel, a Many-to-Many relationship allows for a more flexible and dynamic relationship between two database tables. Many-to-many relationships are common in real-world scenarios, where many items or entities can be related to many other items or entities.
In this blog, we’ll cover the basics of a Many-to-Many relationship in Laravel, including database schema, code, and three real-life examples.
Understanding Many-to-Many Relationship
A Many-to-Many relationship requires a third table to establish the relationship between two tables. For example, let’s consider a real-world scenario where we have two tables: users
and roles
. One user can have multiple roles, and one role can be assigned to multiple users. So, we need a third table role_user
to establish the Many-to-Many relationship between users
and roles
table.
Here’s how the database schema for the three tables would look like:
users table:
- id
- name
- email
- password
roles table:
- id
- name
role_user table:
- id
- role_id
- user_id
In the role_user
table, the role_id
and user_id
columns are foreign keys that reference the id
columns of the roles
and users
tables respectively.
Example 1: A Music Streaming App
Suppose we have a music streaming app where users can follow many artists, and an artist can have many followers. In this scenario, we can use a Many-to-Many relationship between the users
and artists
tables.
Here’s how the database schema would look like:
users table:
- id
- name
- email
- password
artists table:
- id
- name
artist_user table:
- id
- artist_id
- user_id
To retrieve all the artists followed by a particular user, we can define the Many-to-Many relationship in the User
model:
class User extends Model
{
public function artists()
{
return $this->belongsToMany(Artist::class);
}
}
And to retrieve all the followers of an artist, we can define the Many-to-Many relationship in the Artist
model:
class Artist extends Model
{
public function followers()
{
return $this->belongsToMany(User::class);
}
}
We can use eager loading to retrieve all the artists followed by a particular user and all the followers of an artist in a single database query:
$user = User::with('artists')->find(1);
$artist = Artist::with('followers')->find(1);
Example 3: A Job Board App
Suppose we have a recipe sharing app where users can save many recipes, and a recipe can be saved by many users. In this scenario, we can use a Many-to-Many relationship between the users
and recipes
tables.
Here’s how the database schema would look like:
users table:
- id
- name
- email
- password
recipes table:
- id
- name
- ingredients
- instructions
recipe_user table:
- id
- recipe_id
- user_id
To retrieve all the recipes saved by a particular user, we can define the Many-to-Many relationship in the User
model:
class User extends Model
{
public function recipes()
{
return $this->belongsToMany(Recipe::class);
}
}
And to retrieve all the users who saved a particular recipe, we can define the Many-to-Many relationship in the Recipe
model:
class Recipe extends Model
{
public function users()
{
return $this->belongsToMany(User::class);
}
}
We can use eager loading to retrieve all the recipes saved by a particular user and all the users who saved a particular recipe in a single database query:
$user = User::with('recipes')->find(1);
$recipe = Recipe::with('users')->find(1);
Example 2: A Recipe Sharing App
Suppose we have a job board app where companies can post many jobs, and a job can be posted by many companies. In this scenario, we can use a Many-to-Many relationship between the `companies` and `jobs` tables.
Here’s how the database schema would look like:
companies table:
-id
-name
-website
jobs table:
-id
-title
-description
company_job table:
-id
-company_id
-job_id
To retrieve all the jobs posted by a particular company, we can define the Many-to-Many relationship in the `Company` model:
class Company extends Model
{
public function jobs()
{
return $this->belongsToMany(Job::class);
}
}
And to retrieve all the companies that posted a particular job, we can define the Many-to-Many relationship in the `Job` model:
class Job extends Model
{
public function companies()
{
return $this->belongsToMany(Company::class);
}
}
We can use eager loading to retrieve all the jobs posted by a particular company and all the companies that posted a particular job in a single database query:
$company = Company::with('jobs')->find(1);
$job = Job::with('companies')->find(1);
Conclusion:
Many-to-Many relationships are a powerful way to establish dynamic relationships between database tables in Laravel. In this blog, we covered the basics of Many-to-Many relationships, including database schema, code, and three real-life examples. With the help of eager loading, we can efficiently retrieve related data in a single database query.
Popular Post
Table of Contents Introduction: Laravel is a PHP framework that has become quite popular in recent years. One of the
Table of Contents In Laravel, models are the backbone of the application. They act as a bridge between the database
Table of Contents Laravel is a popular PHP framework that is widely used for web application development. One of the
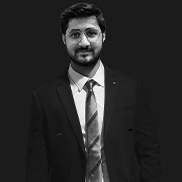
An experienced Code Debugger with a strong track record of identifying and resolving complex software issues. I aim to contribute my analytical skills and expertise in debugging tools to ensure efficient and reliable software operations and deliver high-quality software products that meet customer requirements.
If you found this content valuable, please show your support by following me on Medium, LinkedIn, Twitter and GitHub. Your support will motivate me to create more informative content in the future. Don’t forget to give a clap or share this blog with others if you found it helpful!