Table of Contents In Laravel, many-to-many relationships are established using an intermediate table that holds foreign keys from the related
Laravel’s HasMany Relationship The Key to Managing Complex Database Structures
- Muhammad Waqas
- May 15, 2023
- 5:05 am
- No Comments
Table of Contents
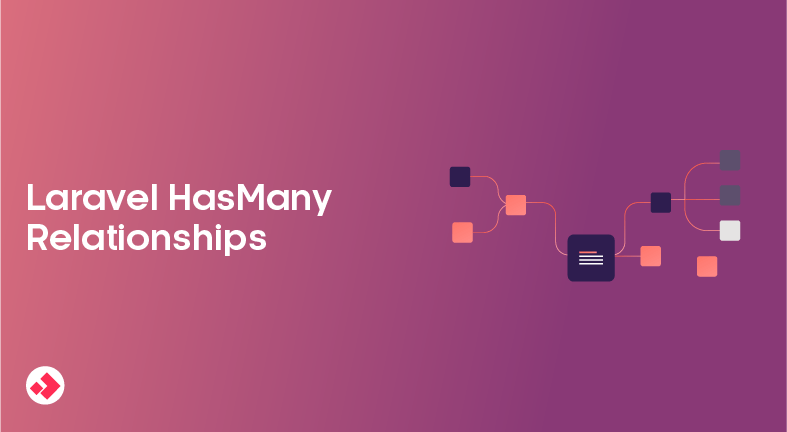
Laravel is a popular PHP web application framework that offers a variety of features and tools to make development easier and more efficient. One of its most powerful features is the ability to manage database relationships using its built-in ORM (Object-Relational Mapping) system, known as Eloquent. In this blog post, we will explore one of the most commonly used relationships in Laravel — the HasMany relationship.
The HasMany relationship is used to define a one-to-many relationship between two database tables. For example, a blog post can have many comments, or a user can have many orders. In Laravel, this relationship can be easily defined and managed using Eloquent.
To illustrate how the HasMany relationship works in Laravel, let’s take a look at some real-life examples.
Example 1: A blog post with comments
Suppose we have a database with two tables — posts
and comments
. Each post
can have many comments
. We can define this relationship in Laravel using the following code:
class Post extends Model
{
public function comments()
{
return $this->hasMany(Comment::class);
}
}
Here, we define a hasMany
relationship between the Post
model and the Comment
model. The comments
method returns a collection of all comments associated with the post.
To retrieve all comments for a particular post, we can simply call the comments
method on the Post
model:
$post = Post::find(1);
$comments = $post->comments;
Example 2: A user with orders
Suppose we have a database with two tables — users
and orders
. Each user
can have many orders
. We can define this relationship in Laravel using the following code:
class User extends Model
{
public function orders()
{
return $this->hasMany(Order::class);
}
}
Here, we define a hasMany
relationship between the User
model and the Order
model. The orders
method returns a collection of all orders associated with the user.
To retrieve all orders for a particular user, we can simply call the orders
method on the User
model:
$user = User::find(1);
$orders = $user->orders;
Example 3: A department with employees
Suppose we have a database with two tables — departments
and employees
. Each department
can have many employees
. We can define this relationship in Laravel using the following code:
class Department extends Model
{
public function employees()
{
return $this->hasMany(Employee::class);
}
}
Here, we define a hasMany
relationship between the Department
model and the Employee
model. The employees
method returns a collection of all employees associated with the department.
To retrieve all employees for a particular department, we can simply call the employees
method on the Department
model:
$department = Department::find(1);
$employees = $department->employees;
In each of these examples, we have defined a HasMany relationship between two database tables using Eloquent. We can easily retrieve related records using the methods generated by Eloquent.
Conclusion
In conclusion, the HasMany relationship is a powerful feature of Laravel’s Eloquent ORM that makes it easy to manage one-to-many relationships in a database. By defining a relationship between two models, we can easily retrieve related records using the methods generated by Eloquent. The examples presented in this blog post show how this relationship can be used in real-life scenarios.
Popular Post
Table of Contents Introduction: Laravel is a PHP framework that has become quite popular in recent years. One of the
Table of Contents In Laravel, models are the backbone of the application. They act as a bridge between the database
Table of Contents Laravel is a popular PHP framework that is widely used for web application development. One of the
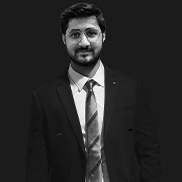
An experienced Code Debugger with a strong track record of identifying and resolving complex software issues. I aim to contribute my analytical skills and expertise in debugging tools to ensure efficient and reliable software operations and deliver high-quality software products that meet customer requirements.
If you found this content valuable, please show your support by following me on Medium, LinkedIn, Twitter and GitHub. Your support will motivate me to create more informative content in the future. Don’t forget to give a clap or share this blog with others if you found it helpful!