Table of Contents In Laravel, many-to-many relationships are established using an intermediate table that holds foreign keys from the related
Exploring the Powerful “Has Many Through” Relationship in Laravel: 3 Real-Life Examples
- Muhammad Waqas
- May 15, 2023
- 5:03 am
- No Comments
Table of Contents
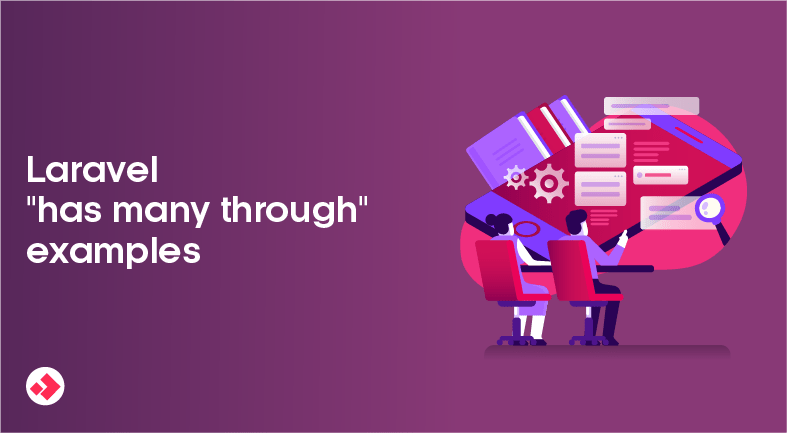
In Laravel, relationships are a crucial aspect of database design and querying. One of the most powerful relationships is the “has many through” relationship, which allows you to retrieve records that belong to a particular model through a third model. This relationship is particularly useful when dealing with complex data structures and allows you to retrieve related data in a single query.
In this blog post, we will explore the “has many through” relationship in Laravel and provide three real-life examples of its usage, along with the code and explanation.
Understanding Has Many Through Relationship
Before we dive into the examples, let’s take a quick look at the “has many through” relationship in Laravel. This relationship is defined by three models: a source model, an intermediate model, and a destination model. The source model has a “has many” relationship with the intermediate model, and the intermediate model has a “has many” relationship with the destination model. The “has many through” relationship allows you to retrieve all the related records of the destination model through the intermediate model.
Here’s an example to make it more clear. Let’s say we have three models: User
, Role
, and Permission
. A User
can have multiple Roles
, and each Role
can have multiple Permissions
. We can define the “has many through” relationship as follows:
class User extends Model
{
public function permissions()
{
return $this->hasManyThrough(Permission::class, Role::class);
}
}
In this example, we’re defining a permissions
method on the User
model, which uses the hasManyThrough
method to retrieve all the Permission
records associated with a User
through their Role
records.
Example 1: E-commerce Application
Let’s take the example of an e-commerce application where we have three models: User
, Order
, and Product
. A User
can have multiple Orders
, and each Order
can have multiple Products
. We can use the “has many through” relationship to retrieve all the Product
records associated with a User
through their Order
records.
class User extends Model
{
public function products()
{
return $this->hasManyThrough(Product::class, Order::class);
}
}
In this example, we’re defining a products
method on the User
model, which uses the hasManyThrough
method to retrieve all the Product
records associated with a User
through their Order
records. We can eager load the products
relationship as follows:
$users = User::with('products')->get();
This will retrieve all the User
records along with their associated Product
records, and it will only execute two queries.
Example 2: Social Media Application
Let’s take the example of a social media application where we have three models: User
, Post
, and Tag
. A User
can have multiple Posts
, and each Post
can have multiple Tags
. We can use the “has many through” relationship to retrieve all the Tag
records associated with a User
through their Post
records.
class User extends Model
{
public function tags()
{
return $this->hasManyThrough(Tag::class, Post::class);
}
}
In this example, we’re defining a tags
method on the User
model, which uses the hasManyThrough
method to retrieve all the Tag
records associated with a User
through their Post
records. We can eager load the tags
relationship as follows:
$users = User::with('tags')->get();
This will retrieve all the User
records along with their associated Tag
records, and it will only execute two queries.
Example 3: Employee Management System
Let’s take the example of an employee management system where we have three models: Department
, Employee
, and Project
. A Department
can have multiple Employees
, and each Employee
can work on multiple Projects
. We can use the “has many through” relationship to retrieve all the Project
records associated with a Department
through their Employee
records.
class Department extends Model
{
public function projects()
{
return $this->hasManyThrough(Project::class, Employee::class);
}
}
In this example, we’re defining a projects
method on the Department
model, which uses the hasManyThrough
method to retrieve all the Project
records associated with a Department
through their Employee
records. We can eager load the projects
relationship as follows:
$departments = Department::with('projects')->get();
This will retrieve all the Department
records along with their associated Project
records, and it will only execute two queries.
Conclusion
In conclusion, the “has many through” relationship is a powerful feature in Laravel that allows you to retrieve related records through a third model. This relationship can be used in a variety of real-life scenarios, such as e-commerce applications, social media applications, and employee management systems. By using eager loading, you can retrieve all the related records in a single query, which can significantly improve the performance of your application.
Popular Post
Table of Contents Introduction: Laravel is a PHP framework that has become quite popular in recent years. One of the
Table of Contents In Laravel, models are the backbone of the application. They act as a bridge between the database
Table of Contents Laravel is a popular PHP framework that is widely used for web application development. One of the
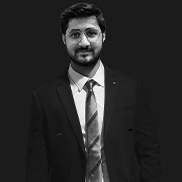
An experienced Code Debugger with a strong track record of identifying and resolving complex software issues. I aim to contribute my analytical skills and expertise in debugging tools to ensure efficient and reliable software operations and deliver high-quality software products that meet customer requirements.
If you found this content valuable, please show your support by following me on Medium, LinkedIn, Twitter and GitHub. Your support will motivate me to create more informative content in the future. Don’t forget to give a clap or share this blog with others if you found it helpful!