Table of Contents In Laravel, many-to-many relationships are established using an intermediate table that holds foreign keys from the related
Creating Test Data in Laravel A Comprehensive Guide to Factories and Seeders
- Muhammad Waqas
- May 15, 2023
- 5:07 am
- No Comments
Table of Contents
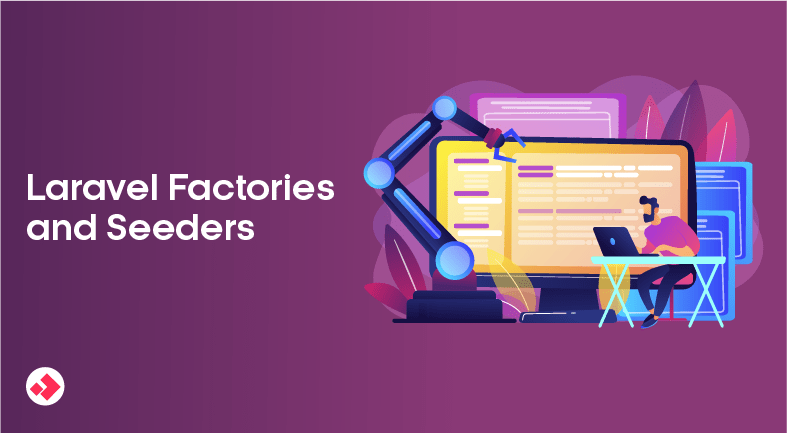
Laravel is a popular PHP web application framework that comes with many features and tools for developers to create efficient and robust web applications. Two of the most commonly used features in Laravel are factories and seeders. In this blog post, we will discuss the basics of factories and seeders in Laravel with proper code examples.
What is a factory in Laravel?
A factory in Laravel is a PHP class that is used to generate fake data for testing purposes. The factory can be used to create instances of any model in your Laravel application, and it generates realistic and random data for the model’s attributes. Factories are particularly useful for creating test data quickly and easily.
Creating a Factory in Laravel
To create a factory in Laravel, you need to use the factory()
helper function. This function takes two arguments – the class name of the model you want to create instances of, and a closure function that defines the data you want to generate for the model’s attributes.
Here is an example of a simple factory for a User
model:
use App\Models\User;
use Illuminate\Database\Eloquent\Factories\Factory;
class UserFactory extends Factory
{
protected $model = User::class;
public function definition()
{
return [
'name' => $this->faker->name(),
'email' => $this->faker->unique()->safeEmail(),
'password' => bcrypt('password'),
];
}
}
In this example, we have created a UserFactory
class that extends the Factory
class provided by Laravel. We have set the $model
property to the User
class, which tells Laravel which model this factory is associated with.
The definition()
method is where we define the data we want to generate for the model’s attributes. In this case, we are generating a random name using the faker
library, a unique and safe email address, and a bcrypt hashed password.
What is a seeder in Laravel?
To create a seeder in Laravel, you need to use the php artisan make:seeder
Artisan command. This command generates a new seeder class in the database/seeders
directory.
Here is an example of a simple seeder that uses the UserFactory
we created earlier to generate test data for the users
table:
use Illuminate\Database\Seeder;
use App\Models\User;
class UserSeeder extends Seeder
{
public function run()
{
User::factory()->count(50)->create();
}
}
In this example, we have created a UserSeeder
class that extends the Seeder
class provided by Laravel. In the run()
method, we are using the UserFactory
to generate 50 instances of the User
model and inserting them into the users
table using the create()
method.
Using Factories and Seeders in Laravel
Now that we have created a factory and a seeder, we can use them to generate test data for our Laravel application. To seed the database with the test data, we need to run the php artisan db:seed
Artisan command. This command will execute all of the seeder classes in the database/seeders
directory.
Here is an example of how to use the UserSeeder
to generate test data for the users
table:
php artisan db:seed --class=UserSeeder
This command will execute the UserSeeder
class and insert 50 instances of the User
model into the users
table in the database.
To use the factory to generate test data in your tests, you can use the create()
method provided by the factory. Here is an example of how to create a new user using the UserFactory
:
$user = User::factory()->create();
In this example, we are using the create()
method provided by the UserFactory
to create a new user instance and save it to the database.
You can also use the make()
method provided by the factory to create a new instance of the model without saving it to the database. This can be useful for testing purposes when you need to generate test data but don’t want to persist it to the database.
$user = User::factory()->make();
In this example, we are using the make()
method provided by the UserFactory
to create a new user instance without saving it to the database.
Conclusion:
Factories and seeders are powerful tools that can save you a lot of time when developing web applications. Factories allow you to generate realistic and random test data for your models, while seeders allow you to populate the database with that test data. By using factories and seeders, you can easily create test data for your Laravel application and ensure that it is functioning as expected.
Popular Post
Table of Contents Introduction: Laravel is a PHP framework that has become quite popular in recent years. One of the
Table of Contents In Laravel, models are the backbone of the application. They act as a bridge between the database
Table of Contents Laravel is a popular PHP framework that is widely used for web application development. One of the
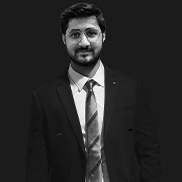
An experienced Code Debugger with a strong track record of identifying and resolving complex software issues. I aim to contribute my analytical skills and expertise in debugging tools to ensure efficient and reliable software operations and deliver high-quality software products that meet customer requirements.
If you found this content valuable, please show your support by following me on Medium, LinkedIn, Twitter and GitHub. Your support will motivate me to create more informative content in the future. Don’t forget to give a clap or share this blog with others if you found it helpful!