Table of Contents In Laravel, many-to-many relationships are established using an intermediate table that holds foreign keys from the related
Code Reuse in Laravel How to Use Traits for Modularity and Efficiency
- Muhammad Waqas
- May 15, 2023
- 5:06 am
- No Comments
Table of Contents
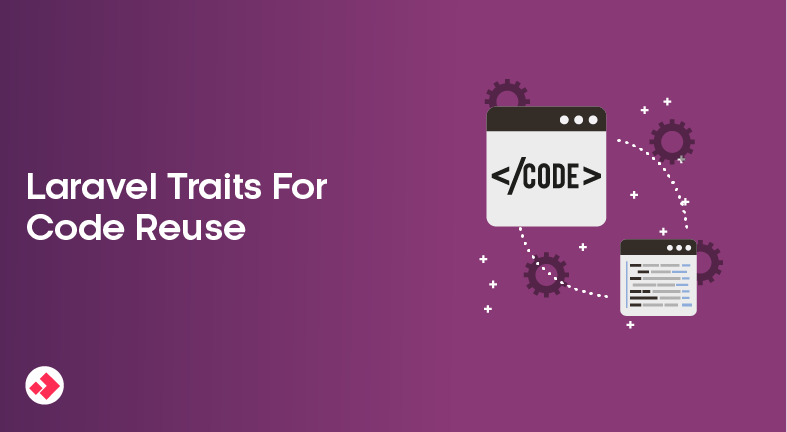
Laravel is a powerful and popular PHP framework that is used for building web applications. One of the features of Laravel is traits, which is a mechanism for code reuse in object-oriented programming. Traits allow developers to reuse code across different classes without the need for inheritance.
In this blog post, we will explore traits in Laravel and provide three examples of how they can be used in practice.
What are Traits?
In object-oriented programming, a trait is a set of methods that can be reused across different classes. Traits are similar to classes in that they define methods, but they cannot be instantiated on their own. Instead, they are intended to be used in conjunction with classes to provide additional functionality.
Traits can be thought of as a way to mix in behavior to a class without creating a new class hierarchy. They can be used to provide functionality that is not present in the class hierarchy, or to override existing functionality.
Example 1: Logging Trait
One common use case for traits is to provide logging functionality to classes. For example, we may want to log every time a particular method is called. We can accomplish this by creating a LogsActivity
trait:
trait LogsActivity {
public function logActivity($message) {
// code to log activity
}
}
We can then use this trait in any class that needs logging functionality:
class UserController {
use LogsActivity;
public function index() {
$this->logActivity('User index page viewed');
// code to display user index page
}
}
Now every time the index()
method is called, the logActivity()
method from the LogsActivity
trait will be executed.
In this example, we are using the “using” method to specify the custom intermediate table model, and the “withPivot” method to include the “status” column in the pivot table.
Example 2: Soft Deletes Trait
Another use case for traits is to provide soft delete functionality to classes. Soft deletes allow records to be marked as deleted without actually removing them from the database. This can be useful for auditing purposes or for restoring deleted records.
Laravel provides a built-in SoftDeletes
trait that can be used in models to enable soft delete functionality:
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\SoftDeletes;
class Post extends Model {
use SoftDeletes;
protected $dates = ['deleted_at'];
}
With this trait enabled, we can now call the delete()
method on a Post
instance and it will be marked as deleted:
$post = Post::find(1);
$post->delete();
We can then retrieve deleted records using the withTrashed()
or onlyTrashed()
methods:
// retrieve all posts, including deleted ones
$posts = Post::withTrashed()->get();
// retrieve only deleted posts
$deletedPosts = Post::onlyTrashed()->get();
Example 3: User Roles Trait
A final example of a trait in Laravel is a UserRoles
trait that can be used to provide role-based access control functionality to user models:
trait UserRoles {
public function isAdmin() {
return $this->role === 'admin';
}
public function isEditor() {
return $this->role === 'editor';
}
}
We can then use this trait in our User
model to check the role of a user:
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
class User extends Authenticatable {
use Notifiable, UserRoles;
// code for user model
}
We can now check the role of a user using the isAdmin()
and isEditor()
methods:
$user = User::find(1);
if ($user->isAdmin()) {
// allow access to admin features
} else if ($user->isEditor()) {
// allow access to editor features
} else {
// restrict access
}
Using traits in this way allows us to easily add and remove functionality from our models without having to modify the class hierarchy.
Conclusion:
In conclusion, traits are a powerful feature of Laravel that can be used to provide code reuse and modularity in object-oriented programming. We have provided three examples of how traits can be used in Laravel: for logging, soft deletes, and user roles. By using traits, we can create cleaner and more modular code that is easier to maintain and extend.
Popular Post
Table of Contents Introduction: Laravel is a PHP framework that has become quite popular in recent years. One of the
Table of Contents In Laravel, models are the backbone of the application. They act as a bridge between the database
Table of Contents Laravel is a popular PHP framework that is widely used for web application development. One of the
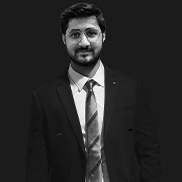
An experienced Code Debugger with a strong track record of identifying and resolving complex software issues. I aim to contribute my analytical skills and expertise in debugging tools to ensure efficient and reliable software operations and deliver high-quality software products that meet customer requirements.
If you found this content valuable, please show your support by following me on Medium, LinkedIn, Twitter and GitHub. Your support will motivate me to create more informative content in the future. Don’t forget to give a clap or share this blog with others if you found it helpful!