Table of Contents In Laravel, many-to-many relationships are established using an intermediate table that holds foreign keys from the related
Translating Text in Laravel Made Easy with TranslateTextHelper and Google Translate Library A Comprehensive Guide
- Muhammad Waqas
- May 15, 2023
- 5:08 am
- No Comments
Table of Contents
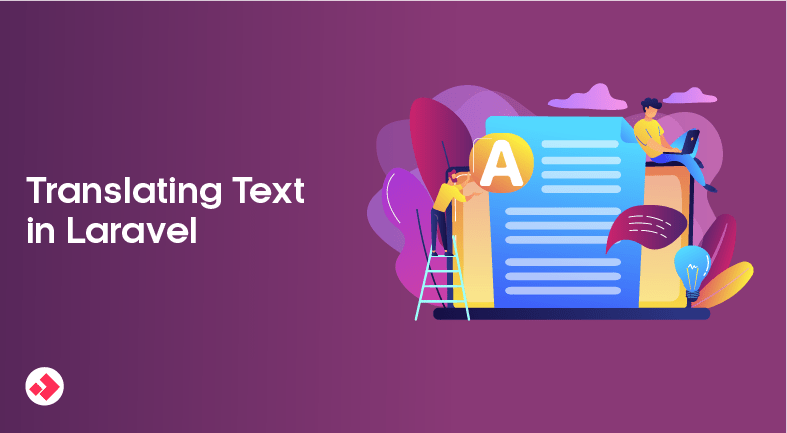
Introduction:
Translation of text is a common requirement for many web applications that need to support users from different regions speaking different languages. In Laravel, we can use third-party libraries to translate text from one language to another. In this blog, we will discuss a helpful code snippet for translating text using the Google Translate PHP library.
Problem Statement:
The need for language translation arises when we want to support users who speak different languages. We may need to translate dynamic content at runtime, such as user-generated content, comments, or messages. The code we are discussing here provides a solution for this problem.
The code we are discussing here is a helper class named TranslateTextHelper
that uses the Google Translate PHP library. The TranslateTextHelper
class has two static variables $source
and $target
, which hold the source and target language codes, respectively. The class provides two methods to set these variables, setSource()
and setTarget()
. The translate()
method takes a string as input and returns the translated string.
Here is the code for the TranslateTextHelper
class:
setSource(self::$source);
$translator->setTarget(self::$target);
$translatedText = $translator->translate($text);
} catch (LargeTextException|RateLimitException|TranslationRequestException $ex) {
Log::error('TranslateTextHelperException', [
'message' => $ex->getMessage(),
]);
}
return $translatedText;
}
}
How to use this code:
To use this code, we need to include the Google Translate PHP library in our Laravel application. We can do this by installing the library using Composer:
composer require stichoza/google-translate-php
After installing the library, we can use the TranslateTextHelper
class to translate text. Here is an example of how to use the TranslateTextHelper
class to translate a string:
use App\Helpers\TranslateTextHelper;
// Set the source and target languages
TranslateTextHelper::setSource('en')->setTarget('ar');
// Translate the text
$translatedText = TranslateTextHelper::translate('Hello, world!');
// Output the translated text
echo $translatedText;
In this example, we first set the source and target languages using the setSource
and setTarget
methods, respectively. Then, we call the translate
method to translate the text. The translated text is stored in the $translatedText
variable, which we then output to the screen.
Note:stichoza/google-translate-php package may work without an API key,it is based on crawling the Google Translate website.
You can find more information about this package by visiting the following link:
https://github.com/Stichoza/google-translate-php
Conclusion:
In conclusion, the TranslateTextHelper
class is a simple and useful tool for translating text from one language to another in your Laravel projects. By using the google-translate-php
library, this class provides a reliable and easy-to-use solution for translating text at runtime.
Credit to Library Owner:
I would like to give credit to the owner of the google-translate-php
library, Stichoza, for creating such a useful tool for PHP developers. Without their hard work and dedication, this class would not be possible. The library can be found at https://github.com/Stichoza/google-translate-php.
Popular Post
Table of Contents Introduction: Laravel is a PHP framework that has become quite popular in recent years. One of the
Table of Contents In Laravel, models are the backbone of the application. They act as a bridge between the database
Table of Contents Laravel is a popular PHP framework that is widely used for web application development. One of the
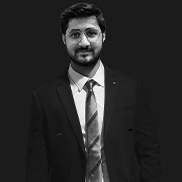
An experienced Code Debugger with a strong track record of identifying and resolving complex software issues. I aim to contribute my analytical skills and expertise in debugging tools to ensure efficient and reliable software operations and deliver high-quality software products that meet customer requirements.
If you found this content valuable, please show your support by following me on Medium, LinkedIn, Twitter and GitHub. Your support will motivate me to create more informative content in the future. Don’t forget to give a clap or share this blog with others if you found it helpful!